Map
Map is a built-in function in python. It is used for transformation of the objects in a list or tuple.
Map function iterates through a list or tuple (iterable objects) implicitly and applies a function in each element.
Returns a map value.
It takes 2 arguments:
- Function to be applied
- Iterable Object
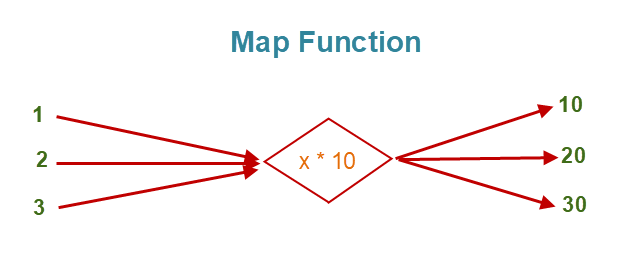
Lets take an example. There is a list of pet animals and birds. We need to convert all the string values in the list to uppercase.
Without using Map:
pets = ['Cat','Dog','Parrot','Ant','Bird']
uppercase = []
for names in pets:
name_upper = names.upper()
uppercase.append(name_upper)
print(uppercase)
['CAT', 'DOG', 'PARROT', 'ANT', 'BIRD']
With Map:
myfriends = ['Cat','Dog','Parrot','Ant','Bird']
uppercase = list(map(str.upper,myfriends))
print(uppercase)
['CAT', 'DOG', 'PARROT', 'ANT', 'BIRD']
In the map function, we sent the first argument as a function str.upper. The second argument is an iterable object on which the function to be applied.
Another example: Lets say we have a list of bill price values. We need to round the values.
floatNum = [4.77,2.33,9.02,10.67,0.66,4.67]
print(floatNum)
intNew = list(map(round,floatNum))
print(intNew)
[4.77, 2.33, 9.02, 10.67, 0.66, 4.67] [5, 2, 9, 11, 1, 5]
Filter
The Filter function applies a boolean function to each item of the iterable object and return the items only if the function returns True for it.
- Init signature: filter(self, /, *args, **kwargs)
- Docstring: filter(function or None, iterable) –> filter object
- Return an iterator yielding those items of iterable for which function(item) is true.
- If function is None, return the items that are true.
- It is similar to map but the only difference is that, filter will return the element of list only if the condition is true.
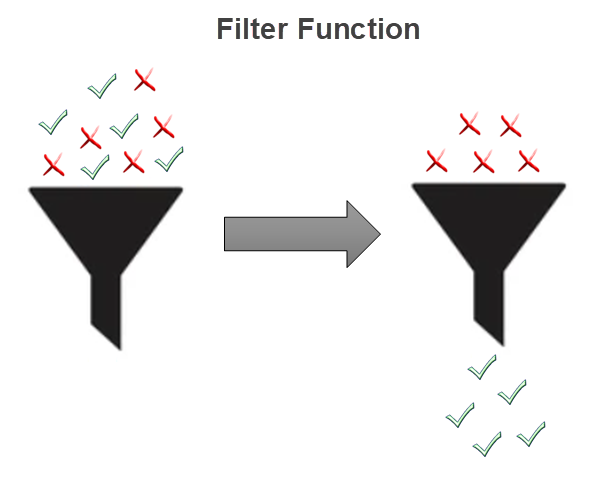
Without Filter:
Lets say we have a list of people’s age. We need to filter only the age that is eligible for voting (> 18).
# Prints the age which is >= 18
#Filter ages without filter() function
age_list = [15,18,45,90,5]
def eligibility_vote(age):
return age >= 18
for age in age_list:
if(eligibility_vote(age)):
print(age)
18 45 90
With Filter Function:
#With Filter
age_list = [15,18,45,90,5]
def eligibility_vote(age):
return age >= 18
list(filter(eligibility_vote, age_list))
[18, 45, 90]
What if the function is not a Boolean function?
It process the function but returns all the elements as it is.
As Filter function is not a transformation function, it will not change the items of the iterable object. It will just filter the items if the function is a Boolean function.
#Filter
age_list = [15,18,45,90,5]
def eligibility_vote_all(age):
if age >= 18:
return age * 10
else:
return age * 10
#Without Filter
for age in age_list:
if(eligibility_vote_all(age)):
print(age)
new_list = list(filter(eligibility_vote_all,age_list))
print(new_list)
15 18 45 90 5 [15, 18, 45, 90, 5]
Another Example:
words = ['Myth','Eat','Cry','Mass']
vowels = ['a','e','i','o','u']
def withVowel(a):
for c in a:
if c in vowels:
return True
return False
list(filter(withVowel,words))
['Eat', 'Mass']
Filter Vs Map In terms of for loops:
- Filter returns the value only if the boolean function returns True.
- Map function applies a function to all the items regardless of the return value of the function and creates a new iterable object with the result.
Map:
list(map(eligibility_vote,age_list))
[False, True, True, True, False]
Filter:
list(filter(eligibility_vote,age_list))
[18, 45, 90]