User Input:
Input(message) function waits for an input from user.
Input function always takes in a string data type value from the user.
x = input("Enter: ")
print(type(x))
print(type(int(x)))
Output:
Enter: 5 <class 'str'> <class 'int'>
Format Output:
There may be situations, where we may need to dynamically place a value in a sub string of a string.
You may think it is easy that we can concatenate strings.
For example: “The patient name is ” + patient_name + “ and age is ” + patient_age
But Python provides a beautiful way where you can even format the patient_name and patient_age to align, pad spaces or any special characters, in case of float values, we can also format decimal places.
The string object’s format function will format the given values in specified formats and place them inside the place holders. {} curly brackets are used as place holders.
output = "The patient's name is {}, {}.".format("firstname","lastname")
print(output)
Output:
The patient's name is firstname, lastname.
print("The patient name is {b:d} and age is {a:d}".format(a=5,b=10))
print("The patient name is {b} and age is {a}".format(a=5,b="test"))
print("The patient name is {1:d} and age is {0:d}".format(5,10))
print("The patient name is {1} and age is {0}".format(5,10))
Output:
The string value 10 is output 5 The string value test is output 5 The string value 10 is output 5 The string value 10 is output 5
Named Arguments:
We can name the arguments, so that no need to worry about the order of arguments.
#Named arguments
print("The patient name is {b} and age is {a}".format(a=5,b="test"))
#Indexed arguments
print("The patient name is {1} and age is {0}".format(5.14,"test"))
Output:
The patient name is test and age is 5 The patient name is test and age is 5.14
Placeholders:
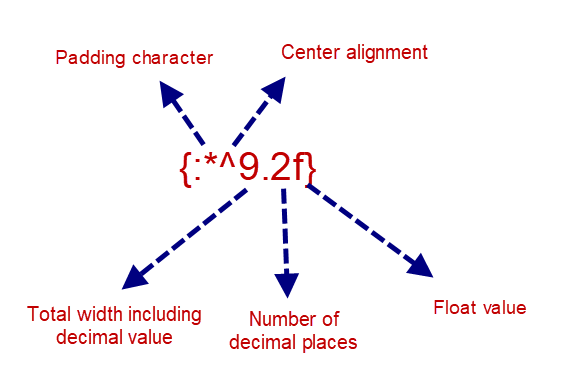
We can also change the display format of the values using place holder curly brackets.
print("The string value {:*^6} is output".format("a"))
# * - padding, if not mentioned empty space
# ^ - center, < - left, > right
# 6 - any number specifies the number of character space to use as minimum width
Output:
The string value **a*** is output
After the colon symbol “:”, we can mention the format options.
The first character (*) will be used for padding.
If only the first character is used, Value Error will be displayed as “Unknown format code ‘*’ “.
The second character (^) specifies the alignment.
^ – Center; < – Left; > – Right
The third character is the minimum width space that should be used un the placeholder. If the input value is longer than this value, then this will be overridden by the length of the input.
Due to the number as 6, the space taken for that place holder is 6.
In the 6 spaces, text “a” is centered as ^ symbol is used.
The remaining places were filled with * as mentioned.
Decimal places format:
print("The string value {:*^15.2f} is output".format(15206))
Output:
The string value ***15206.00**** is output
Now it is mentioned as 15.2f for width.
The .2f means 2 decimal places. 15 means totally 15 characters long.
If the given input is non-numeric, then “ValueError: Unknown format code ‘f’ for object of type ‘str'” will occur.