Loops are block of statements that will be repeatedly executed until the condition is true.
Python has 2 types of loops:
Type #1: While Loop:
execute until given condition is true
n = 5
while (n > 0):
print(n)
n = n-1
Output:
5 4 3 2 1
Type #2: For Loop:
execute for all the elements in a range or list.
For loop can be used with any type of iterable objects easily. For example: List, Tuple, Set, Dict, String, range etc.
list1 = [5,10,2,24,10]
for i in list1:
print(i)
Output:
5 10 2 24 10
Break and Continue Statement:
Break statement is used to terminate the loop. i.e., once the break statement is encountered, the remaining code will not be executed and the control will be out if the loop. All the remaining iterations will be skipped. The statement follows next to the loop will be executed.
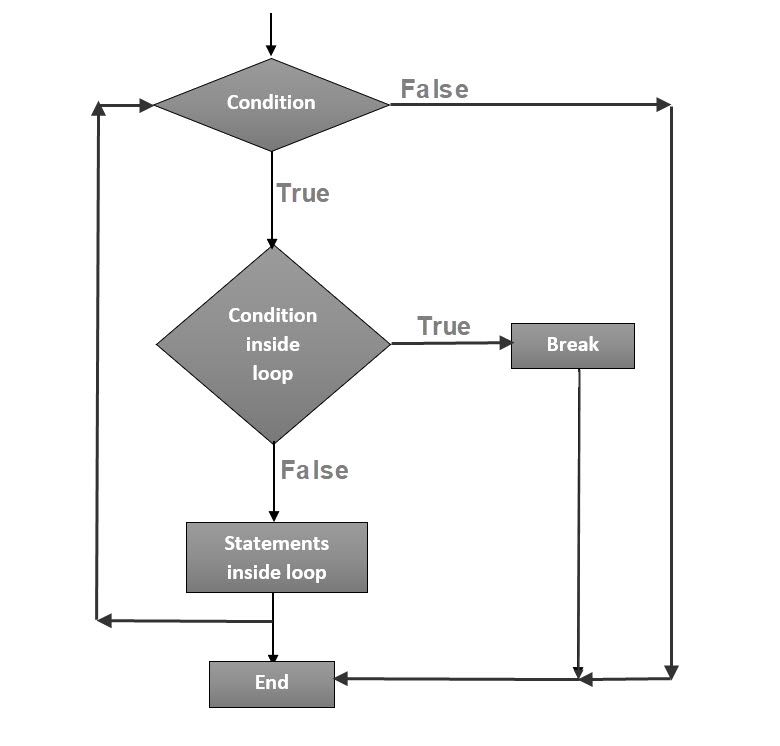
num_list = [2,4,6,8,10]
for i in num_list:
if(i > 5):
break;
print(i)
print("Exit")
Output:
2 4 Exit
In the above code, the break statement is encountered once the condition is satisfied. So the value 6 is encountered, the condition gets passed and the break statement is executed. Thus the remaining lines of code and remaining iterations are skipped. The print statement which follows the loop is executed.
The same flow is applicable for while loop too.
Continue statement is used to skip the remaining statements of the current iteration. The entire loop will not be terminated. The control will flow with the next item of the iteration.
num_list = [1,2,3,4,5]
for i in num_list:
if(i % 2 == 0):
continue;
print(i)
print("Exit")
Output:
1 3 5 Exit
In the above example, the continue statement will be encountered only if the value is divisible by 2. So the continue statement is used here to skip all the even numbers. The loop will still run for all the odd numbers.
Else Block in Loops:
In Python, Else block can be used in while and for loops.
Else block will be executed once the looping condition gets failed. So the last statement executed in loop will be the else block.
If there is a break in a loop, as the remaining code and iterations skipped, the else statement will also be skipped. So, the else statement will be executed only if there is no termination in loops.
Else block in While Loop:
n = 5
while (n > 0):
print(n)
n = n-1
else:
print("Else Block")
Output:
5 4 3 2 1 Else Block
Else block in For loop:
num_list = [5,10,24]
for i in num_list:
print(i)
else:
print("Else Block")
Output:
5 10 24 Else Block