Python Functions are block of statements that can be called to do a set of operations whenever needed.
It can be called multiple times anywhere from a program that has access to the function.
It can return the value to the called statement [optional]
It can have parameters to pass into the function. Parameters can have default values.
A function should be defined before the call to it.
Lets create a simple python function to say Hello:
def greetings():
print("Hello there...")
greetings()
Hello there...
In the above code, we have created a function just to say Hello.
Whenever, wherever you want to print this statement, you can just call this function. That will print the Hello statement.
Parameterized Function:
Lets improve this function to display the user name such as “Hello Rohan…”. But we do not know the name of the user when defining the function itself. The name will be known at the place from where we call this function.
For that, we need to mention the place holder and need to tell the program what to print when the function is called.
To do this, First, we need to name the placeholder. This placeholder is called as parameters. Second, we need to give the name information from where it is called. We send the values while calling the function with parameters.
def greetings(name):
print("Hello " + name + "...")
greetings("Rohan")
Hello Rohan...
Now, the above function is having a parameter “name”.
What if the function is called without parameters? When a parameter is defined but it was not mentioned while it is called, the below error will be occurred.
--------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-2-cdae29c445f4> in <module> 4 5 greetings("Rohan") ----> 6 greetings() TypeError: greetings() missing 1 required positional argument: 'name'
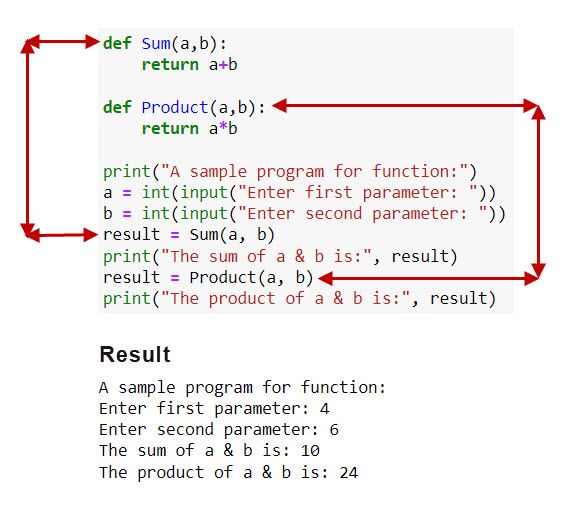
Default Parameters:
What if some users are anonymous? At that time the display message will not be as expected. It will be like “Hello …”.
Also, there is a possibility of missing the parameters in a function call. But it may not be expected to raise an error.
In python, we can also set a default value if no parameters are given. Using this, even if the parameter is missed, the compiler will not bother about this and set the default value.
def greet(name = "there..."):
print("Hello " + name)
greet("Rohan")
greet()
Hello Rohan Hello there...
Function Return Values:
A function can also return a value from where it is called.
def Sum(a,b):
return a+b
print("Sum of 10 & 5 is", Sum(10,5))
Sum And Difference of 10 & 5 is 15
Return Multiple Values:
Python can also return multiple values to the calling statement. When multiple values are returned, the values will be returned as a single tuple with multiple values in it.
def SumAndDiff(a,b):
return a+b,a-b,a*b
returned = SumAndDiff(10,5)
print("Returned value type:",type(returned))
print("Sum And Difference of 10 & 5 is", returned)
Returned value type: <class 'tuple'> Sum And Difference of 10 & 5 is (15, 5, 50)
Type Hints in Functions:
Type hints are useful to know the data type of the parameters. For example, in the previous example, the parameters a & b must be numeric. Otherwise the type related exceptions must be occur.
Even though we can write validations, when a new developer use an age old function in a new functionality, the developer may not know the expected data type of the parameter. At that time, a string value may be sent in place of a numeric values. This may cause functionality issues. To avoid this, we can give a hint to the developers to mention the type of the parameters.
def multiply(a, b):
return a * b
def addition(a, b):
return a + b
Here we have defined 2 functions. When using + sign in strings, it will not throw exception. But it will change the functionality from addition to concatenation.
print(addition(5,6))
print(addition("5","6"))
11 56
print(multiply(5,6))
print(multiply(5,"6"))
print(multiply("5","6"))
30 66666
--------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-8-051e65134284> in <module> 1 print(multiply(5,6)) ----> 2 print(multiply("5","6")) <ipython-input-7-9af0c4484b3c> in multiply(a, b) 1 def multiply(a, b): ----> 2 return a * b TypeError: can't multiply sequence by non-int of type 'str'
If any one of the value is numeric say n, then the string will be repeated for n number of times.
When trying to multiply 2 string values, the TypeError will occur.
def hinted_multiply(a: int, b: int) -> int:
return a * b
This type hint will help the developer to send only the integer values. This is just a hint and it will not force the compiler to only allow the specified data type. Still the Dynamic Typing will work.
print(hinted_multiply(5,6))
print(hinted_multiply(5,"6"))
print(hinted_multiply("5","6"))
30 66666
--------------------------------------------------------------------------- TypeError Traceback (most recent call last) <ipython-input-14-a1b0df3ffaad> in <module> 1 print(hinted_multiply(5,6)) 2 print(hinted_multiply(5,"6")) ----> 3 print(hinted_multiply("5","6")) <ipython-input-10-783afba02a73> in hinted_multiply(a, b) 3 4 def hinted_multiply(a: int, b: int) -> int: ----> 5 return a * b TypeError: can't multiply sequence by non-int of type 'str'
Other Exceptions in Functions:
When calling a function, it should have been already defined. Otherwise, NameError will be occurred as the name of the function is not recognized.
printing()
def printing():
print("Printing Test.")
--------------------------------------------------------------------------- NameError Traceback (most recent call last) <ipython-input-1-552a6b420ba5> in <module> ----> 1 printing() 2 3 def printing(): 4 print("Printing Test.") NameError: name 'printing' is not defined