range() function is introduced in Python3, in Python2 it is xrange().
Range can be used to generate a sequence of numbers.
Range will not return direct sequence. It will return a range object of immutable numbers.
Later we need to either iterate through the object or convert it into any data structure like list.
Minimum # of arguments is 1 and maximum is 3.
Syntax:
range(start, stop[, step])
Arguments:
Stop:
Required.
An integer number specifying at which position to stop (not included).
Stop number will not be included. The sequence will stop before that number.
Start:
Optional only when step is not provided. If step is provided, then start becomes a required parameter. As Range function will not support named parameters, the first parameter will be taken as Start. When step is used,
An integer number specifying at which position to start.
By default, starts from 0.
Step:
Optional.
An integer number specifying the incrementation.
By default, increments by 1.
Example:
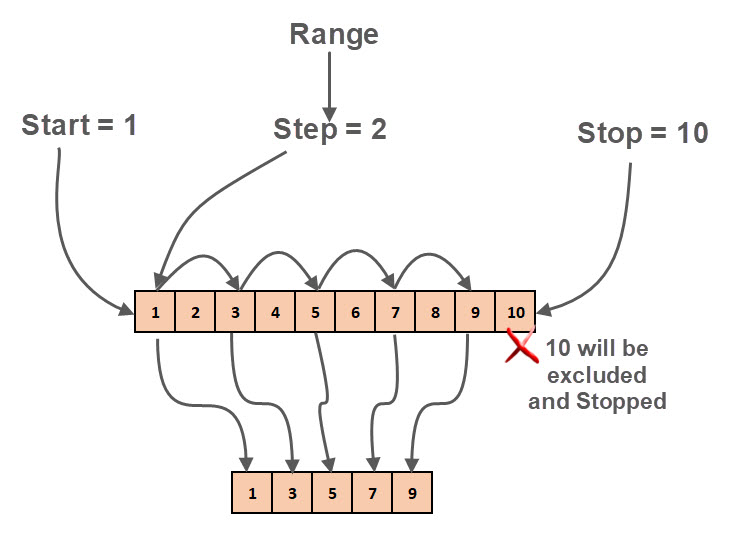
x = list(range(1, 10, 2))
print(x)
Output:
[1, 3, 5, 7, 9]
range_obj = range(1,5)
print(type(range_obj))
print(range_obj)
Output:
<class 'range'> range(1, 5)
for item in range_obj:
print(item)
Output:
1 2 3 4
Convert Range to List:
x = list(range(5, 10))
print(x)
Output:
[5, 6, 7, 8, 9]