In our previous post A Tip A Day – Python Tip #7: OpenCV – CV2: imread() and resize(), we have explored a simple image and its pixel values.
Why divide image by 255?
The pixel values can range from 0 to 256. Each number represents a color code.
When using the image as it is and passing through a Deep Neural Network, the computation of high numeric values may become more complex.
To reduce this we can normalize the values to range from 0 to 1.
In this way, the numbers will be small and the computation becomes easier and faster.
As the pixel values range from 0 to 256, apart from 0 the range is 255. So dividing all the values by 255 will convert it to range from 0 to 1.
import cv2
import matplotlib.pyplot as plt
##Read image
image_path="4.png"
img=cv2.imread(image_path,0)
plt.imshow(img, cmap="gray")
Output:
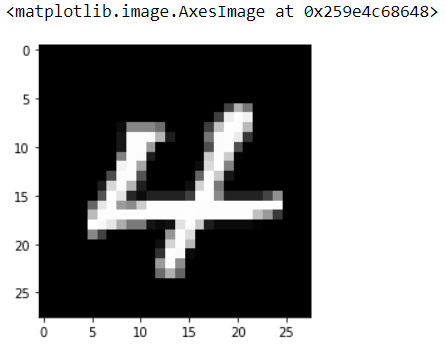
lets take a look at one of the white pizel’s value.
img[17,14]
Output:
253
I gave it a try to visualize the array values using seaborn heatmap and below is the result.
import seaborn as sns
plt.figure(figsize=(30,30))
sns.heatmap(img, annot= True, cmap="binary")
Output:
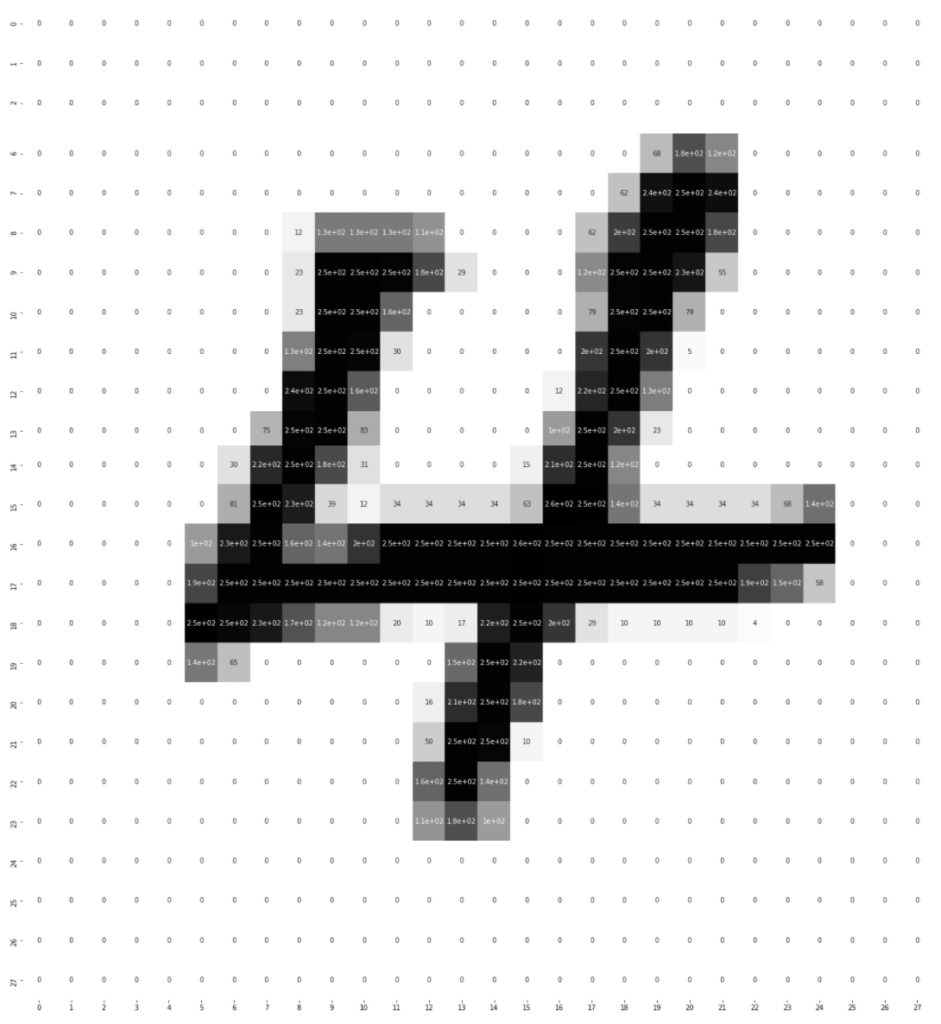
In the above output the values range from 0 to 256.
Normalization:
import seaborn as sns
img = img/255
plt.figure(figsize=(30,30))
sns.heatmap(img, annot= True, cmap="binary")
Output:
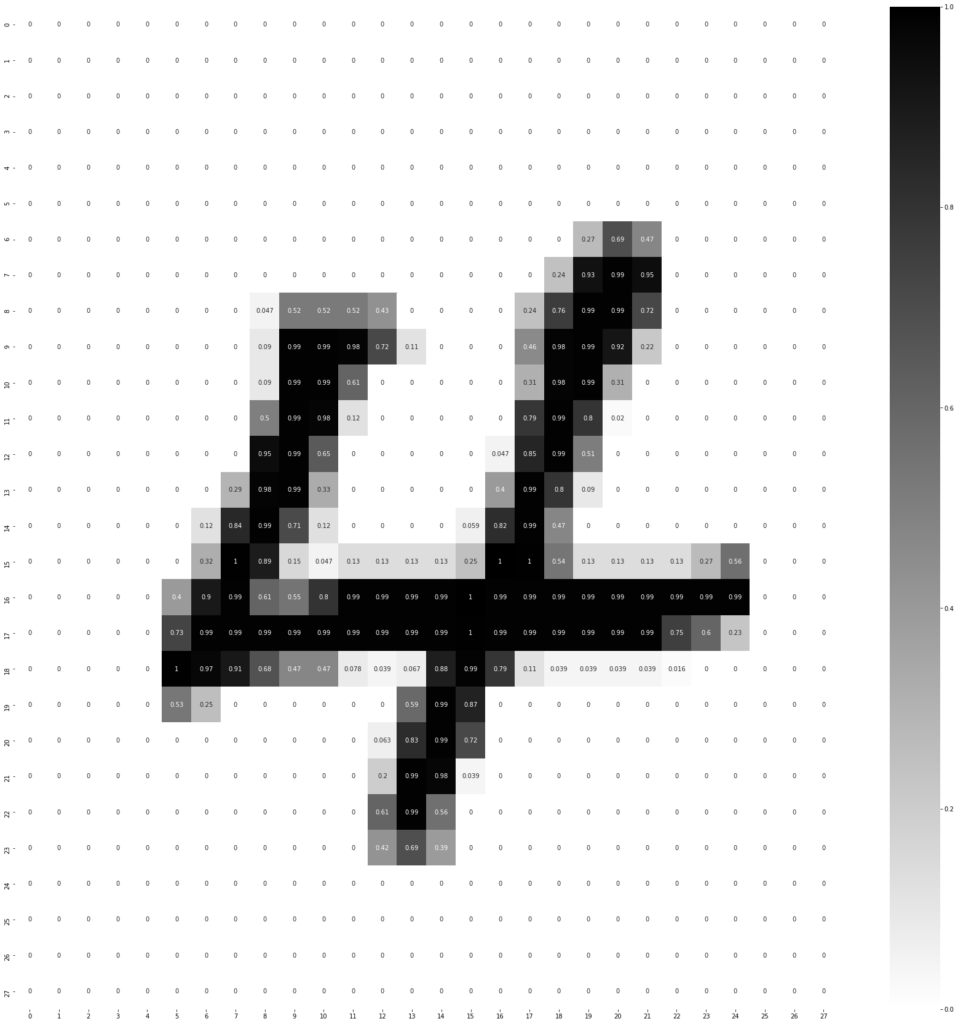
In this result, you can see that pixels that make the strokes in “4” image are 0.93 or 0.99 or near by values.
Hope you can understand why and how we do normalize simple images.
Lets explore more about images in upcoming posts.
More interesting Python tips:
A Tip A Day – Python Tip #6 – Pandas Merge
A Tip A Day – Python Tip #5 – Pandas Concat & Append