There may be situations, where we may need to dynamically place a value in a sub string of a string.
You may think it is easy that we can concatenate strings.
For example: “The patient name is ” + patient_name + “ and age is ” + patient_age
But Python provides a beautiful way where you can even format the patient_name and patient_age to align, pad spaces or any special characters, in case of float values, we can also format decimal places.
The string object’s format function will format the given values in specified formats and place them inside the place holders. {} curly brackets are used as place holders.
1. How to use format:
output = "The patient's name is {}, {}.".format("firstname","lastname")
print(output)
The patient’s name is firstname, lastname.
2. Indexed or Named placeholders:
#Named arguments
print("The patient name is {b} and age is {a}".format(a=5,b="test"))
#Indexed arguments
print("The patient name is {1} and age is {0}".format(5,"test"))
The patient name is test and age is 5
The patient name is test and age is 5
3. Alignment of string:
The placeholders also accept another argument to align the text or number.
Syntax: {index/name : formatting characters}
For number formatting:
- A number to provide the width.
- % – Provides the value as percentage
print("The patient name is {1} and age is {0:%}".format(5.14,"test"))
The patient name is test and age is 514.000000%
String Formatting:
- Any special character to fill the padding space
- < – to align left (or) > – to align right (or) ^ – center
- Any number for minimum width
print("The string value {:*^6} is output".format("a"))
# * - padding, if not mentioned, empty space will be used
# ^ - center
# 6 - any number specifies the number of character space to use as minimum width
The string value **a*** is output
Float value formatting:
Adding a decimal point and the character “f” specifies the expected value is float.
Even thought the given value is a whole number “.00” will be added if “.2f” is specified.
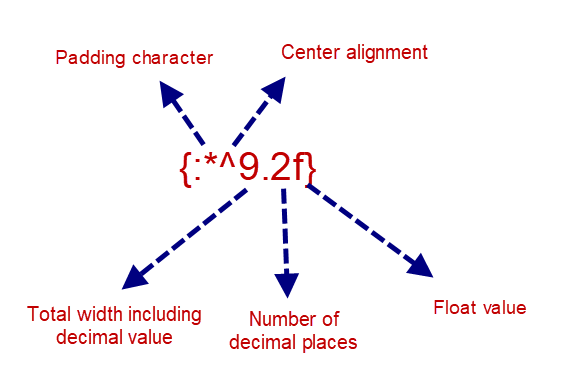
print("The string value {:*^3.2f} is output".format(15.206))
print("The string value {:*^3.2f} is output".format(15206))
print("The string value {:*^15.2f} is output".format(15206))
The string value 15.21 is output
The string value 15206.00 is output
The string value ***15206.00**** is output
Specifying “f” but sending a string value will throw an error.
print("The string value {:*^15.2f} is output".format("15206"))
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-24-09127640ef53> in <module>
----> 1 print("The string value {:*^15.2f} is output".format("15206"))
ValueError: Unknown format code 'f' for object of type 'str'
Thats it!
This post is a bit long for tips.
However I hope you enjoying learning formatting.
Lets explore more about Python in future tips.
More interesting Python tips:
A Tip A Day – Python Tip #6 – Pandas Merge
A Tip A Day – Python Tip #5 – Pandas Concat & Append