In this article, we will see how to join 2 Numpy arrays using built-in funcitons.
Numpy – Joining two numpy arrays
- stack — Joins arrays with given axis element by element
- hstack — Extends horizontally
- vstack — Extends vertically
Stack — Joins arrays with given axis element by element
- Both input arrays should be in same dimension/shape
- Axis parameter in Stack works as dimension here instead of horizontal/vertical manner.
- If Axis is 0, then it will join by first dimension
- If Axis is 1, the it will join by second dimension
- The maximum dimension that we can mention is dimension of input arrays (say n) + 1.
- If axis is given above n + 1, then “out of bounds for array of dimension” exception will be thrown
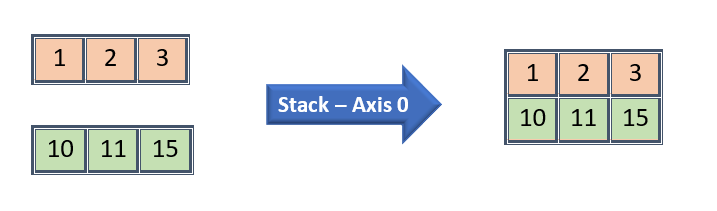
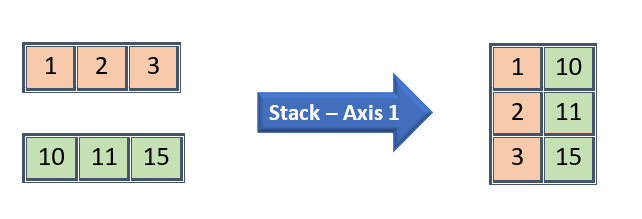
arr1 = np.array([1,5,2,10])
arr2 = np.array([10,50,20,100])
print(arr1)
print(arr2)
Output:
[ 1 5 2 10]
[ 10 50 20 100]
Stack by First Dimension:
[ 1 5 2 10]
[ 10 50 20 100]
Output:
array([[ 1, 5, 2, 10],
[ 10, 50, 20, 100]])
Stack by Second Dimension:
arr3 = np.stack((arr1, arr2), axis = 1)
Output:
array([[ 1, 10],
[ 5, 50],
[ 2, 20],
[ 10, 100]])
HStack — Stacks horizontally
- This function does not work with axis. It extends first array by second array Horizontally
- As it extends horizontally, both the arrays should have same number of rows else Value Error will be returned.
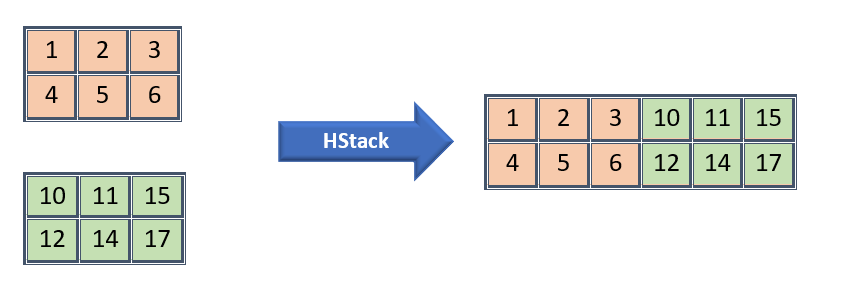
HStack for 1D Arrays:
arr1 = np.array([1,5,2,10])
arr2 = np.array([10,50,20,100])
np.hstack((arr1, arr2))
Output:
array([ 1, 5, 2, 10, 10, 50, 20, 100])
HStack for 2D Arrays:
arr1 = np.array([[1,2,3],[4,5,6]])
arr2 = np.array([[10,20,30],[40,50,60]])
np.hstack((arr1, arr2))
Output:
array([[ 1, 2, 3, 10, 20, 30],
[ 4, 5, 6, 40, 50, 60]])
VStack — Stacks vertically
- This function does not work with axis. It extends first array by second array Vertically
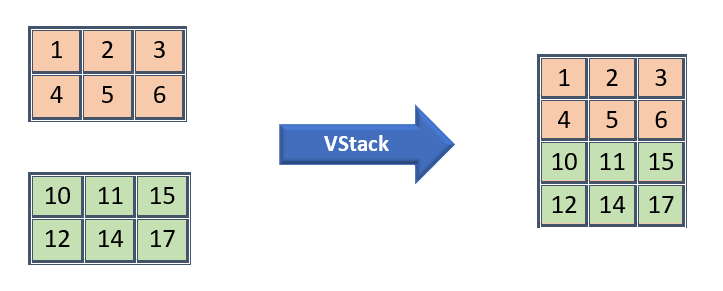
VStack by 2D Arrays:
arr1 = np.array([[1,2,3],[4,5,6]])
arr2 = np.array([[10,20,30],[40,50,60]])
np.vstack((arr1, arr2))
Output:
array([[ 1, 2, 3],
[ 4, 5, 6],
[10, 20, 30],
[40, 50, 60]])
Conclusion:
In this article we experimented 1D and 2D arrays for stacking.
I will be adding another post with more demonstration for 3D arrays as it is little tricky to understand.
If you like this article and support me, just click the heart icon!!!
Subscribe to our site to get notified for new post.
Happy Programming!!!
Like to support? Just click the heart icon ❤️.